Introduction
The Stitch Connect JavaScript Client is a library for integrating Stitch’s data source creation and configuration workflows seamlessly into your web application. Using a pop-up window, end-users can:
- Create a source of a particular type, such as Marketo or Salesforce
- Authorize an existing source
- Run a connection check for an existing source and discover its schema
- Select streams (tables) to replicate for an existing source
- Edit an existing source
Data sources and connection steps
Stitch data sources require a unique sequence of connection steps specific to the source type
to be fully configured.
When a user is sent to a particular step using the JavaScript client, the user will also be prompted to complete any successive steps to complete configuration of the source.
For example: When the addSource
function is used, the user will be prompted to first add the data source. The user will next be directed to authorize the source and select the streams they want to replicate.
Versioning
As of July 22, 2024, the current version of the JavaScript client is version 1.x.x.
Refer to the Releases page in the Stitch JS GitHub repo to for updated release packages.
Installation
Visit the Stitch JS GitHub repo and download the latest release.
In your application, add this <script>
tag just before the closing <body>
tag of the page(s) you want it to run on:
<script type="text/javascript" src="<FILE_PATH>/stitch-client.umd.min.js"></script>
Make sure to replace <FILE_PATH>
with the directory location of the stitch-client.umd.min.js
file.
Error codes
Code | Response text | Description |
AppClosedPrematurelyError |
|
The user closed the Stitch app before successfully completing the task. |
SourceNotFoundError |
|
The provided source ID is invalid. |
UnknownSourceTypeError |
|
The provided source |
UpgradeEphemeralTokenError |
|
There was an issue with updating the session’s ephemeral token. |
Functions
The JavaScript client supports the functions listed below. All of the functions expect an options
object as the only argument and return a Promise
.
addSource(options)
Initiates the creation of a source in the user’s Stitch client account.
Arguments
The addSource
function expects an options
object as the only argument. This object must contain all parameters marked as REQUIRED:
type
STRING REQUIRED |
The source type. For example: |
ephemeral_token
STRING OPTIONAL |
The token used to automatically log the user into the Stitch client account. Retrieved by creating a session using the Connect API’s Create a Session endpoint. If the token is not provided, the user may be prompted to sign into their Stitch account. The ephemeral token expires one hour after generation. When specified as an argument in a Connect JavaScript function, the ephemeral token is exchanged for a session token, creating a temporary Stitch session for the user. The session expires once terminated or 12 hours after its creation. |
default_streams
OBJECT OPTIONAL |
Sets the default selections for the data structures (tables) to be replicated during the source integration setup. Should be an object of the form
Additionally, note that:
|
Example usage
The code below will create a HubSpot integration (type: platform.hubspot
) in the user’s Stitch account, with the campaigns
and companies
tables pre-selected for replication.
Stitch.addSource({
"type": "platform.hubspot",
"ephemeral_token": "<EPHEMERAL_TOKEN>",
"default_streams":{
"campaigns": true,
"companies": true
}
}).then((result) => {
console.log(`Integration created, type=${result.type}, id=${result.id}`);
}).catch((error) => {
console.log("Integration not created.", error);
});
Stitch.js will send the user to Stitch and open the Integration Settings page, where the user can configure the integration.
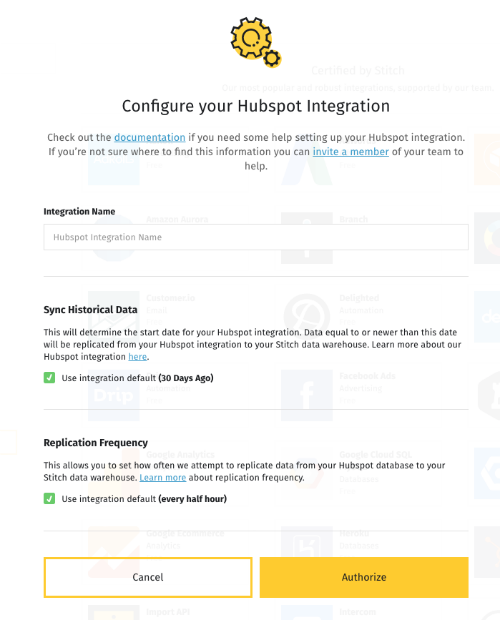
authorizeSource(options)
Sends the user to Stitch, which will redirect to the third-party to complete an OAuth handshake.
Arguments
The authorizeSource
function expects an options
object as the only argument. This object must contain all parameters marked as REQUIRED:
id
INTEGER REQUIRED |
The unique identifier for the source. For example: |
ephemeral_token
STRING OPTIONAL |
The token used to automatically log the user into the Stitch client account. Retrieved by creating a session using the Connect API’s Create a Session endpoint. If the token is not provided, the user may be prompted to sign into their Stitch account. The ephemeral token expires one hour after generation. When specified as an argument in a Connect JavaScript function, the ephemeral token is exchanged for a session token, creating a temporary Stitch session for the user. The session expires once terminated or 12 hours after its creation. |
default_streams
OBJECT OPTIONAL |
Sets the default selections for the data structures (tables) to be replicated during the source integration setup. Should be an object of the form
Additionally, note that:
|
Example usage
The code below will first send the user to Stitch and then re-direct to the appropriate third-party to complete an OAuth handshake.
Stitch.authorizeSource({
"id": 45612,
"ephemeral_token": "<EPHEMERAL_TOKEN>"
}).then((result) => {
console.log(`Integration created, type=${result.type}, id=${result.id}`);
}).catch((error) => {
console.log("Integration not created.", error);
});
Stitch.js will send the user to Stitch and then re-direct to the appropriate third-party to complete an OAuth handshake.
In this example, the source being authorized is HubSpot (type: platform.hubspot
).
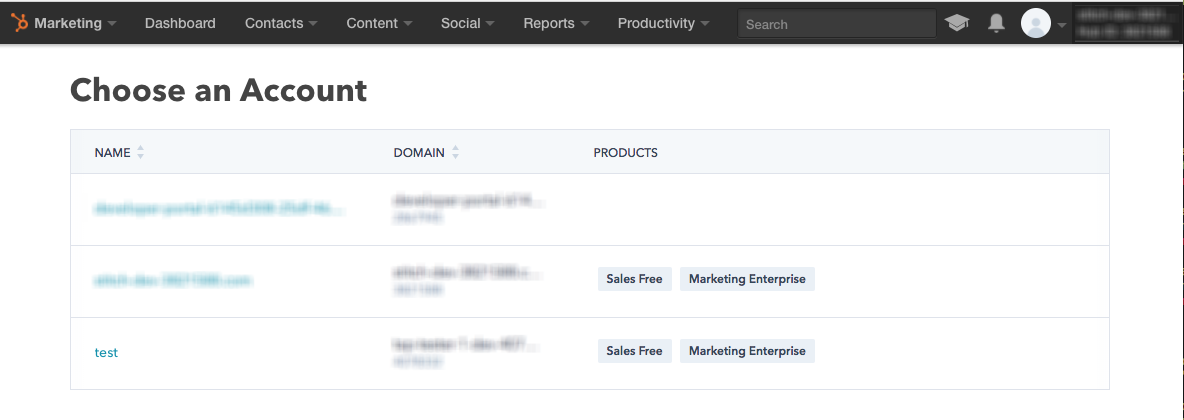
displayDiscoveryOutputForSource(options)
Checks the source’s connection and discovers its schema.
Arguments
The displayDiscoveryOutputForSource
function expects an options
object as the only argument. This object must contain all parameters marked as REQUIRED:
id
INTEGER REQUIRED |
The unique identifier for the source. For example: |
discovery_job_name
STRING REQUIRED |
When a source is updated, Stitch will run a connection check to test the source’s connection parameters and discover its schema. This value is the name of the job that should be displayed. To initiate a connection check, use the Update a Source endpoint. The |
ephemeral_token
STRING OPTIONAL |
The token used to automatically log the user into the Stitch client account. Retrieved by creating a session using the Connect API’s Create a Session endpoint. If the token is not provided, the user may be prompted to sign into their Stitch account. The ephemeral token expires one hour after generation. When specified as an argument in a Connect JavaScript function, the ephemeral token is exchanged for a session token, creating a temporary Stitch session for the user. The session expires once terminated or 12 hours after its creation. |
default_streams
OBJECT OPTIONAL |
Sets the default selections for the data structures (tables) to be replicated during the source integration setup. Should be an object of the form
Additionally, note that:
|
Example usage
The code below will run a connection check, discover the schema for source 45612
, and output the results.
Stitch.displayDiscoveryOutputForSource({
"id": 45612,
"discovery_job_name": "1234-45612-4567891234-checks"
}).then((result) => {
console.log(`Integration created, type=${result.type}, id=${result.id}`);
}).catch((error) => {
console.log("Integration not created.", error);
});
Stitch.js will run a connection check and output the source’s schema. The example below is for source platform.hubspot
.
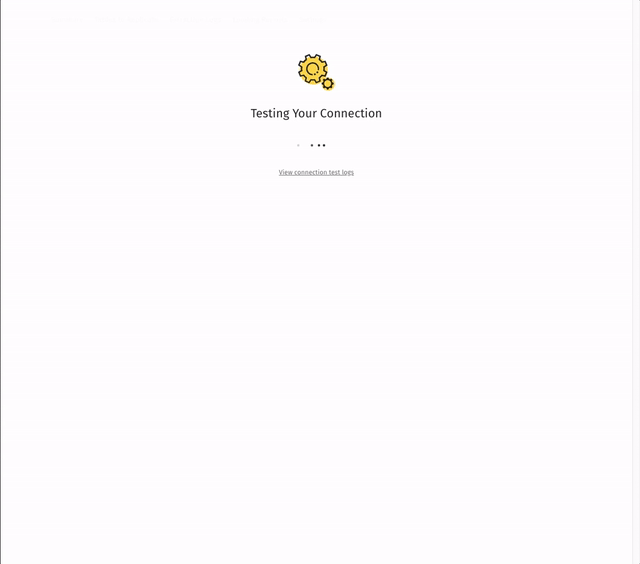
selectStreamsForSource(options)
Selects the tables and fields that should be replicated from the source.
Arguments
The selectStreamsForSource
function expects an options
object as the only argument. This object must contain all parameters marked as REQUIRED:
id
INTEGER REQUIRED |
The unique identifier for the source. For example: |
ephemeral_token
STRING OPTIONAL |
The token used to automatically log the user into the Stitch client account. Retrieved by creating a session using the Connect API’s Create a Session endpoint. If the token is not provided, the user may be prompted to sign into their Stitch account. The ephemeral token expires one hour after generation. When specified as an argument in a Connect JavaScript function, the ephemeral token is exchanged for a session token, creating a temporary Stitch session for the user. The session expires once terminated or 12 hours after its creation. |
default_streams
OBJECT OPTIONAL |
Sets the default selections for the data structures (tables) to be replicated during the source integration setup. Should be an object of the form
Additionally, note that:
|
Example usage
The code below will prompt the user to select the streams (tables) they want to replicate for source 45612
.
Stitch.selectStreamsForSource({
"id": 45612,
"ephemeral_token": "<EPHEMERAL_TOKEN>"
}).then((result) => {
console.log(`Integration created, type=${result.type}, id=${result.id}`);
}).catch((error) => {
console.log("Integration not created.", error);
});
Stitch.js will display the streams available for replication. The example below lists the streams for source platform.hubspot
.
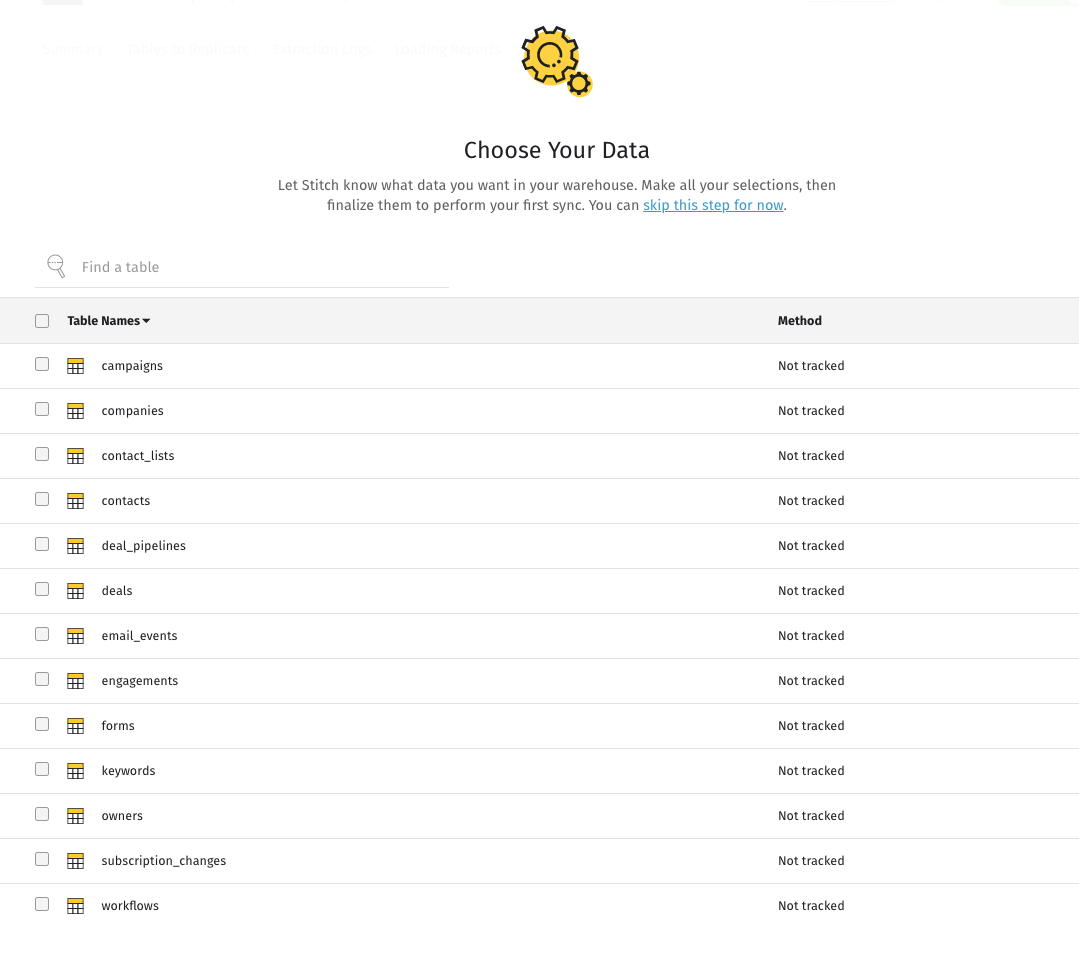
editSource(options)
Edits the source form and settings.
Arguments
The editSource
function expects an options
object as the only argument. This object must contain all parameters marked as REQUIRED:
id
INTEGER REQUIRED |
The unique identifier for the source. For example: |
ephemeral_token
STRING OPTIONAL |
The token used to automatically log the user into the Stitch client account. Retrieved by creating a session using the Connect API’s Create a Session endpoint. If the token is not provided, the user may be prompted to sign into their Stitch account. The ephemeral token expires one hour after generation. When specified as an argument in a Connect JavaScript function, the ephemeral token is exchanged for a session token, creating a temporary Stitch session for the user. The session expires once terminated or 12 hours after its creation. |
default_streams
OBJECT OPTIONAL |
Sets the default selections for the data structures (tables) to be replicated during the source integration setup. Should be an object of the form
Additionally, note that:
|
Example usage
The code below will send the user to Stitch and open the Integration Settings page for source 45612
, where the source’s settings can be updated.
Stitch.editSource({
"id": 45612,
"ephemeral_token": "<EPHEMERAL_TOKEN>"
}).then((result) => {
console.log(`Source updated, type=${result.type}, id=${result.id}`);
}).catch((error) => {
console.log("Editing source failed.", error);
});
Stitch.js will display the Integration Settings page for the source, where the user can update the source’s configuration settings.
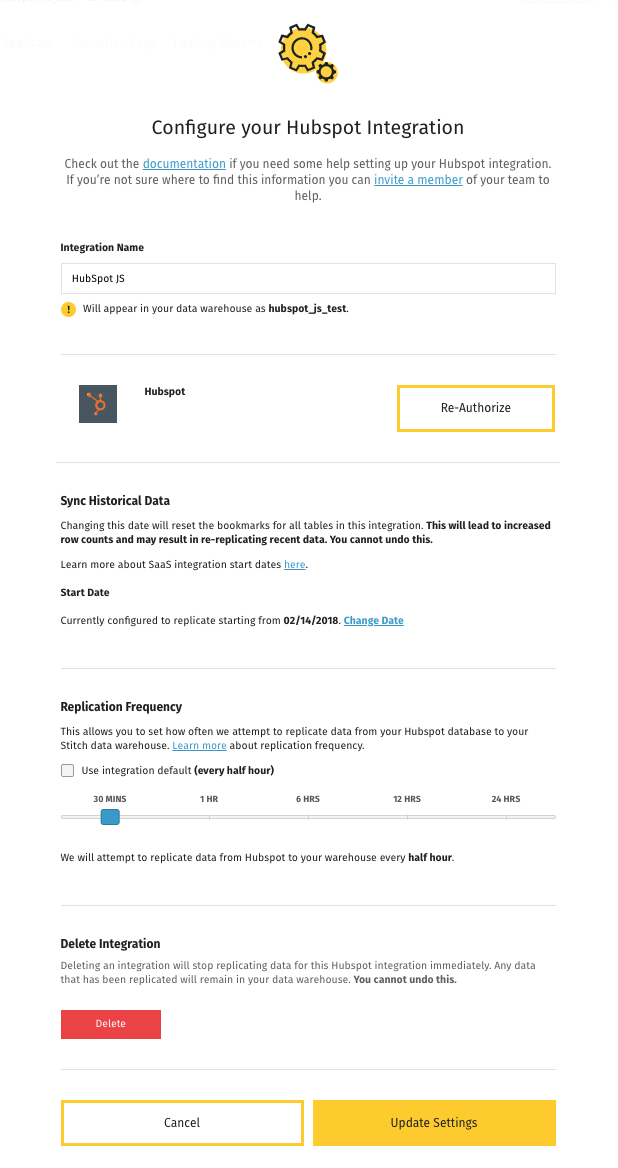